Sustainable Software Development: Green Coding Practices
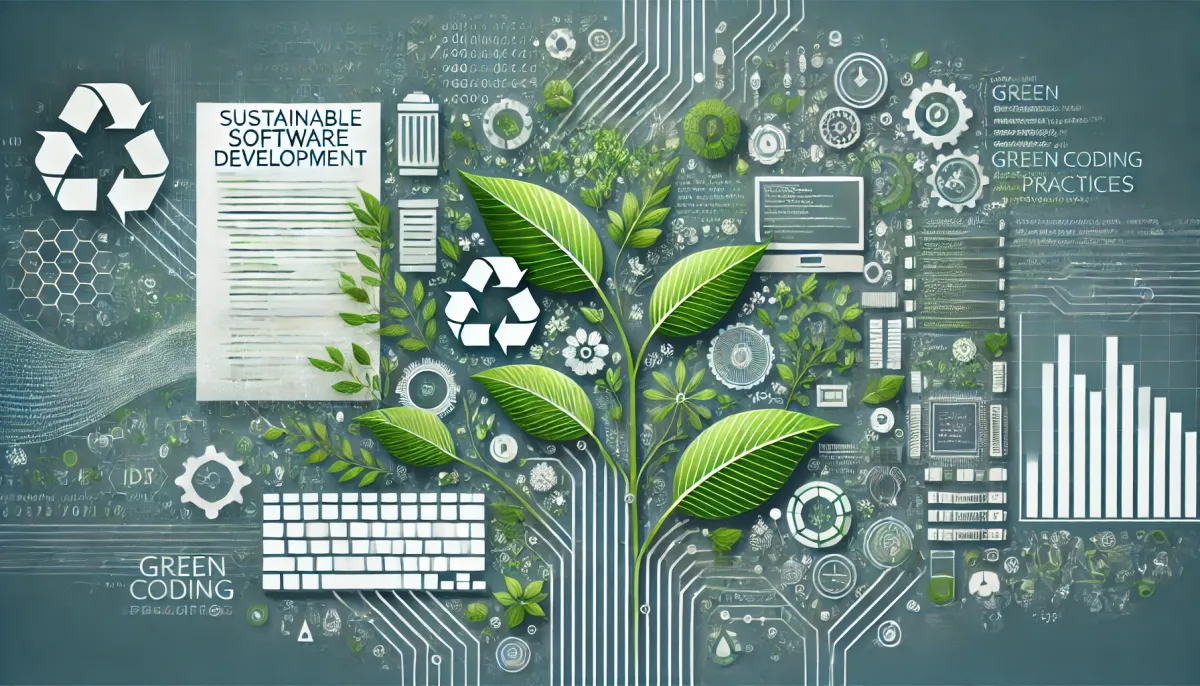
1. Introduction
Did you know that the information and communication technology (ICT) sector is responsible for approximately 2% of global carbon emissions? That's roughly equivalent to the aviation industry's entire carbon footprint. As our reliance on digital technologies continues to grow, so does the environmental impact of the software we create and use daily.
Enter sustainable software development, a paradigm shift in how we approach coding and software design. This concept revolves around creating efficient, long-lasting, and environmentally friendly software solutions. By adopting green coding practices, developers can significantly reduce the energy consumption and carbon footprint of their applications.
In this post, we'll explore the environmental impact of software, delve into the principles of green coding, and provide practical techniques for writing more energy-efficient code. We'll also discuss tools for measuring and improving code efficiency, green hosting practices, and the business case for sustainable software development. Let's dive in and discover how we can make our digital world a little greener, one line of code at a time.
2. The Environmental Impact of Software
When we think about environmental pollution, images of smokestacks and plastic waste often come to mind. However, the digital world has its own form of pollution that's less visible but equally impactful: digital pollution.
Software contributes to energy consumption and carbon emissions in several ways:
- Data Centers: The servers running our applications consume vast amounts of electricity, both for operation and cooling.
- Network Infrastructure: Transferring data across the internet requires energy at every step.
- End-User Devices: The devices running our software, from smartphones to desktop computers, consume energy based on how efficiently our applications run.
Some energy-intensive software practices include:
- Inefficient algorithms that require more processing power than necessary
- Excessive data transfer and storage
- Always-on applications that consume resources even when not actively used
- Unoptimized queries that strain databases and increase response times
For example, a study by the University of Bristol found that watching online videos accounts for nearly 1% of global emissions. This isn't just due to the content itself, but also because of inefficient video processing algorithms and unnecessary high-quality streaming when lower resolutions would suffice.
By understanding these impacts, we can start to see why sustainable software development is crucial for our planet's future.
3. Principles of Green Coding
Green coding is built on four fundamental principles:
Efficiency
Writing code that uses minimal resources is at the heart of green coding. This means choosing appropriate algorithms, data structures, and coding patterns that accomplish the task with the least amount of computational power.
Optimization
Improving existing code to reduce resource usage is an ongoing process. Regular code reviews and refactoring sessions should include considerations for energy efficiency.
Longevity
Creating software that remains functional and efficient over time reduces the need for constant updates and replacements. This includes writing maintainable code and designing flexible architectures that can adapt to changing requirements without complete rewrites.
Measurability
You can't improve what you don't measure. Tracking and reducing energy consumption should be a key performance indicator for software projects, alongside traditional metrics like speed and reliability.
By adhering to these principles, developers can create software that not only performs well but also minimizes its environmental impact.
4. Practical Green Coding Techniques
Let's explore some practical techniques for implementing green coding principles:
Efficient Algorithms and Data Structures
Choosing the right algorithm or data structure can dramatically affect your application's energy consumption. For example, consider the difference between a bubble sort and a quicksort for large datasets:
# Inefficient bubble sort
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
# More efficient quicksort
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
The quicksort algorithm is generally much more efficient for large datasets, resulting in fewer operations and less energy consumption.
Minimizing Network Calls and Data Transfer
Reducing the amount of data transferred over the network can significantly decrease energy consumption. Techniques include:
- Implementing effective caching strategies
- Using compression for data transfers
- Batching API requests instead of making multiple small calls
Here's a simple example of batching API requests in JavaScript:
// Inefficient: Multiple API calls
async function fetchUserData(userIds) {
const userData = [];
for (const id of userIds) {
const response = await fetch(`/api/users/${id}`);
userData.push(await response.json());
}
return userData;
}
// More efficient: Single batched API call
async function fetchUserDataBatched(userIds) {
const response = await fetch('/api/users', {
method: 'POST',
body: JSON.stringify({ ids: userIds }),
});
return response.json();
}
Optimizing Database Queries
Efficient database queries can significantly reduce server load and energy consumption. Key practices include:
- Using appropriate indexes
- Avoiding N+1 query problems
- Implementing database-level caching
Here's an example of an optimized query using an ORM like SQLAlchemy in Python:
# Inefficient: N+1 query problem
def get_users_with_posts():
users = session.query(User).all()
for user in users:
user.posts # This triggers a separate query for each user
# More efficient: Single query with join
def get_users_with_posts_optimized():
return session.query(User).options(joinedload(User.posts)).all()
Implementing Lazy Loading and Efficient Caching Strategies
Lazy loading can significantly reduce initial load times and resource usage by only loading data when it's needed. Here's a simple example in JavaScript:
// Eager loading
import { heavyFunction } from './heavyModule';
// Lazy loading
const heavyFunction = () => import('./heavyModule').then(module => module.heavyFunction);
For caching, consider using service workers for web applications or implementing an efficient caching layer in your backend services.
Using Appropriate Data Types and Avoiding Unnecessary Operations
Choosing the right data type can have a significant impact on memory usage and processing efficiency. For example, in Python:
# Less efficient: Using a float for integer values
account_balance = 1000.0
# More efficient: Using an integer
account_balance = 1000
# Avoid unnecessary string operations
name = "Alice"
greeting = "Hello, " + name + "!" # Less efficient
greeting = f"Hello, {name}!" # More efficient
By implementing these techniques, developers can significantly reduce the energy consumption of their applications without sacrificing functionality or user experience.
5. Tools for Measuring and Improving Code Efficiency
To effectively implement green coding practices, it's crucial to measure and analyze your code's performance and energy consumption. Here are some valuable tools:
Profiling Tools
Most programming languages have built-in or third-party profiling tools. For example:
- Python: cProfile, line_profiler
- JavaScript: Chrome DevTools Performance tab, Node.js --prof flag
- Java: JProfiler, YourKit
Energy Consumption Measurement Tools
- Intel Power Gadget: Measures power usage on Intel processors
- PowerTOP: Linux tool for diagnosing power consumption issues
- Android Studio Energy Profiler: For measuring energy use in Android apps
Static Code Analysis Tools
These tools can help identify inefficiencies without running the code:
- SonarQube: Supports multiple languages and can be configured to check for energy efficiency
- ESLint (for JavaScript): Can be configured with rules to catch potential inefficiencies
- Pylint (for Python): Includes some checks that can impact energy efficiency
Tutorial: Using Python's cProfile
Let's walk through a brief tutorial on how to use cProfile to identify performance bottlenecks:
import cProfile
import pstats
from pstats import SortKey
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
def main():
result = fibonacci(30)
print(f"The 30th Fibonacci number is: {result}")
cProfile.run('main()', 'output.dat')
with open('output_time.txt', 'w') as f:
p = pstats.Stats('output.dat', stream=f)
p.sort_stats(SortKey.TIME).print_stats()
This script will run the main()
function, which calculates the 30th Fibonacci number, and output profiling information to output_time.txt
. The profiling data will show you which functions are taking the most time, helping you identify areas for optimization.
By regularly using these tools, you can ensure your code remains efficient and energy-friendly as it evolves.
6. Green Hosting and Deployment Practices
The way we deploy and host our applications can have a significant impact on their overall energy consumption. Here are some practices to consider:
Choosing Eco-Friendly Cloud Providers
Not all cloud providers are created equal when it comes to sustainability. Look for providers that:
- Use renewable energy sources for their data centers
- Have high Power Usage Effectiveness (PUE) ratings
- Offer carbon-neutral hosting options
Some cloud providers known for their sustainability efforts include Google Cloud Platform, Microsoft Azure, and Amazon Web Services (which has pledged to be 100% renewable by 2025).
Implementing Efficient Scaling Strategies
Proper scaling ensures you're not running more servers than necessary:
- Use auto-scaling to match resources to actual demand
- Implement predictive scaling based on historical data
- Consider using serverless architectures for sporadic workloads
Here's a simple example of setting up auto-scaling in AWS using Terraform:
resource "aws_autoscaling_group" "example" {
availability_zones = ["us-west-2a", "us-west-2b", "us-west-2c"]
desired_capacity = 1
max_size = 3
min_size = 1
target_group_arns = [aws_lb_target_group.example.arn]
health_check_type = "ELB"
launch_template {
id = aws_launch_template.example.id
version = "$Latest"
}
}
resource "aws_autoscaling_policy" "example" {
name = "example-cpu-policy"
autoscaling_group_name = aws_autoscaling_group.example.name
policy_type = "TargetTrackingScaling"
target_tracking_configuration {
predefined_metric_specification {
predefined_metric_type = "ASGAverageCPUUtilization"
}
target_value = 40.0
}
}
This configuration sets up an auto-scaling group that will maintain CPU utilization around 40%, scaling up or down as needed.
Utilizing Containerization and Serverless Architectures Effectively
Containers and serverless functions can lead to more efficient resource utilization:
- Use container orchestration tools like Kubernetes to optimize resource allocation
- Leverage serverless platforms for event-driven workloads to avoid idle resources
For example, here's a simple AWS Lambda function in Python that could replace a constantly running server for infrequent tasks:
import json
def lambda_handler(event, context):
# Process event data
result = process_data(event['data'])
return {
'statusCode': 200,
'body': json.dumps('Processed result: ' + str(result))
}
def process_data(data):
# Your processing logic here
return data.upper()
By adopting these green hosting and deployment practices, you can significantly reduce the energy footprint of your applications in production.
7. The Business Case for Green Coding
While the environmental benefits of green coding are clear, there are also compelling business reasons to adopt these practices:
Cost Savings
Efficient code translates directly to reduced infrastructure costs:
- Lower cloud computing bills due to decreased resource usage
- Reduced energy costs for on-premises servers
- Longer hardware lifespans due to decreased load
Meeting Consumer Demand
Increasingly, consumers are factoring sustainability into their purchasing decisions:
- 66% of consumers consider sustainability when making a purchase (IBM Institute for Business Value, 2020)
- Companies with strong sustainability practices outperform their peers financially (Harvard Business Review, 2016)
Regulatory Compliance
As awareness of digital pollution grows, regulations are emerging:
- The EU's Green Deal includes provisions for digital sustainability
- Some countries are implementing carbon taxes that could affect data centers
Competitive Advantage
Being an early adopter of green coding practices can set your company apart:
- Improved brand image and reputation
- Attraction of environmentally conscious talent
- Potential for innovation in energy-efficient technologies
By framing green coding as not just an environmental initiative but a business imperative, companies can justify the investment in time and resources required to implement these practices.
8. Challenges and Considerations
While the benefits of green coding are significant, there are challenges to consider:
Balancing Performance, Features, and Sustainability
Sometimes, the most energy-efficient solution may not be the fastest or most feature-rich. Developers and product managers need to carefully weigh these trade-offs.
Team-Wide Commitment and Education
Implementing green coding practices requires buy-in from the entire development team. This may necessitate:
- Training programs on sustainable development practices
- Updates to coding standards and review processes
- New performance metrics that include energy efficiency
Overcoming Perceptions
There's a common misconception that sustainable coding practices are time-consuming or costly to implement. In reality, many green coding practices align with general best practices for efficient, high-quality code.
To address these challenges, consider:
- Gradually introducing green coding practices
- Showcasing quick wins to build momentum
- Integrating sustainability into existing development processes rather than treating it as a separate initiative
9. Future Trends in Sustainable Software Development
The field of sustainable software development is rapidly evolving. Some emerging trends to watch include:
Artificial Intelligence for Code Optimization
AI-powered tools are being developed to automatically analyze and optimize code for energy efficiency. These tools could revolutionize how we approach green coding.
Energy-Aware Programming Languages
New programming languages and frameworks are being designed with energy efficiency as a core principle, rather than an afterthought.
Edge Computing
As edge computing grows, we may see a shift towards more distributed, energy-efficient computing models that reduce the load on centralized data centers.
Quantum Computing
While still in its early stages, quantum computing has the potential to solve certain types of problems with significantly less energy than classical computers.
Green Software Engineering Certifications
We may see the emergence of professional certifications specifically for sustainable software development, similar to existing certifications for energy-efficient building design.
As these trends develop, the practice of green coding is likely to become more sophisticated, automated, and integral to the software development process.
10. Conclusion
Sustainable software development is no longer just a nice-to-have – it's becoming a crucial aspect of responsible and forward-thinking software engineering. By adopting green coding practices, we can significantly reduce the environmental impact of our digital world while often improving performance and reducing costs.
As developers, we have the power to shape the future of technology. Let's commit to making that future a sustainable one. Start by implementing some of the techniques discussed in this post in your next project. Experiment with profiling tools to identify energy hotspots in your code. Consider sustainability in your architectural decisions.
Remember, every line of efficient code, every optimized query, and every thoughtful design decision contributes to a greener digital ecosystem. The journey towards fully sustainable software development may be long, but it's one we must undertake for the sake of our planet and future generations.
Let's code not just for today, but for a sustainable tomorrow. The time for green coding is now – are you ready to take up the challenge?